Ajouter et supprimer des éléments d’un JComboBox Java
JComboBox fait partie du package Java Swing. JComboBox hérite de la classe JComponent. JComboBox affiche un menu contextuel qui affiche une liste et l’utilisateur peut sélectionner une option dans cette liste spécifiée. Dans ce tutoriel nous allons découvrir comment ajouter et supprimer des éléments d’un JComboBox.
Exemple:
import javax.swing.*; import java.awt.*; import java.awt.event.*; class ComboBoxExemple extends JFrame implements ItemListener, ActionListener { // frame static JFrame frame; // combobox static JComboBox combobox; // label static JLabel l1, l2; // textfield static JTextField text; public static void main(String[] args) { // créer un nouvea frame frame = new JFrame("Ajouter et supprimer des items"); // créer un objet ComboBoxExemple obj = new ComboBoxExemple(); // tableau de chaînes contenant des langages String langs[] = { "Java", "PHP", "Python", "C++", "Ruby" }; // créer une case à cocher combobox = new JComboBox(langs); // create textfield text = new JTextField(10); // create add and remove buttons JButton b1 = new JButton("Ajouter"); JButton b2 = new JButton("Supprimer"); // add action listener b1.addActionListener(obj); b2.addActionListener(obj); // ajouter ItemListener combobox.addItemListener(obj); // créer des étiquettes l1 = new JLabel("Quel est votre langage prefere? "); l2 = new JLabel("[Java]"); // définir la couleur du texte l2.setForeground(Color.blue); // créer un nouveau panneau JPanel p = new JPanel(); // ajouter combobox, textfield, button et labels au panneau p.add(l1); p.add(combobox); p.add(l2); p.add(text); p.add(b1); p.add(b2); // ajouter le panneau au frame frame.add(p); // définir la taille du frame frame.setSize(400, 200); frame.setLayout(new GridLayout(2, 1)); frame.show(); } // si le bouton est appuyé public void actionPerformed(ActionEvent e) { String str = e.getActionCommand(); if (str.equals("Ajouter")) { combobox.addItem(text.getText()); } else { combobox.removeItem(text.getText()); } } public void itemStateChanged(ItemEvent e) { // si l'état du combobox est modifiée if (e.getSource() == combobox) { l2.setText(" ["+combobox.getSelectedItem()+"]"); } } }
Sortie:
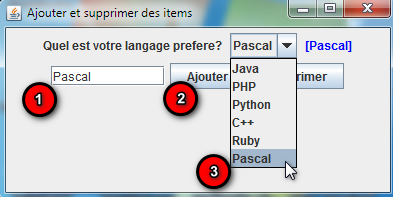